How to add Tailwind CSS 3 and its official plugins to your Vue.js 3 project
John Champ on Feb 16, 2022 · Updated on Mar 22, 2024 · 3 min read
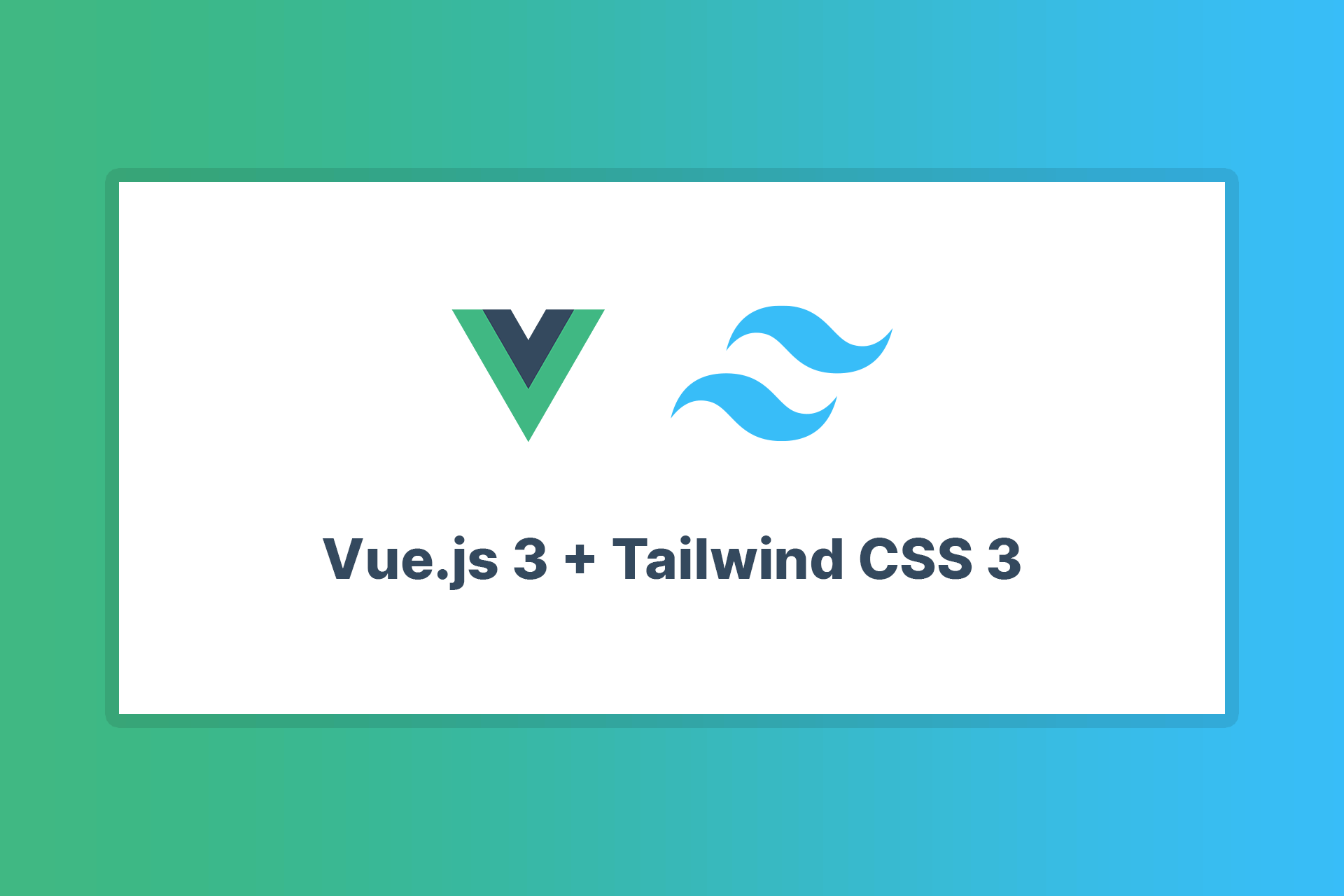
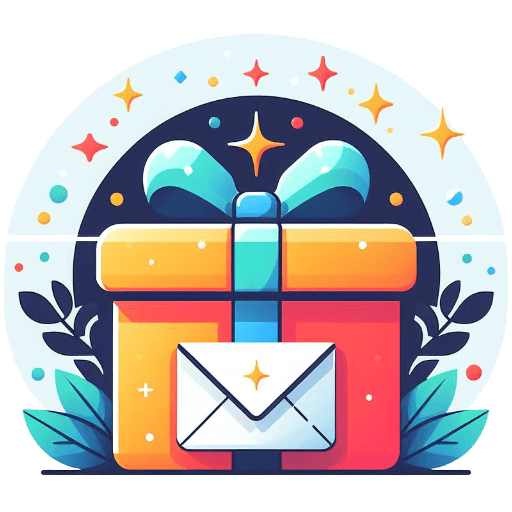
Unlock 15+ exclusive templates
Every two weeks or so, we share work insights, project updates, interesting links or exclusive free templates and offers. Subscribe now and we’ll also send you a custom UI Design Kit we put together just for you!
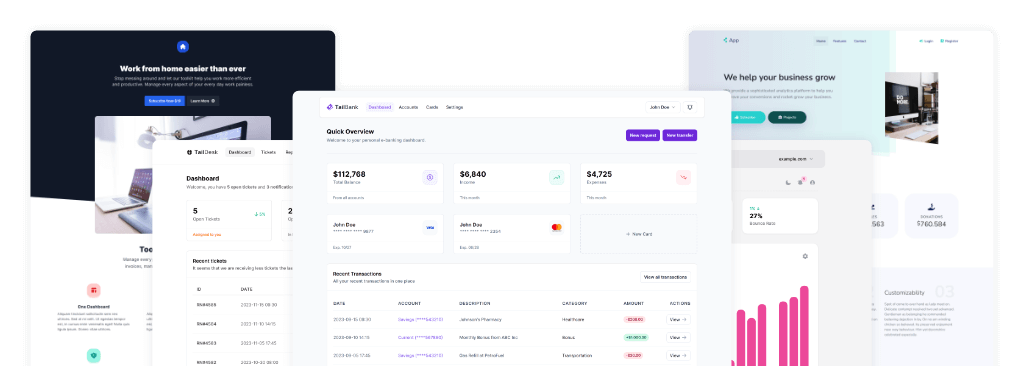